When asked which programming language to learn first - especially for kids - my usual answer is JavaScript [1]. Nothing beats the direct feedback you get from code that's able to paint things on the screen, without having to install anything.
One library that makes it a particularly pleasant process is p5.js, which was created specifically for this educational purpose. I've had good experience teaching kids basic programming using p5.js. Here's a simple example of what I mean:
(You should see some circles moving on the canvas; click on the canvas to add more)
Even though this demo is so trivial, it has many of the elements of creating simple games - colorful stuff is drawn on the screen, things are moving around according to simple physical laws, and there's interactivity!
Here's the entire code required to implement this with p5.js:
let circles = [];
function setup() {
createCanvas(400, 400);
for (let i = 0; i < 5; i++) {
circles.push(randomCircleAtPos(
width / 2 + random(-100, 100),
height / 2 + random(-100, 100)));
}
}
function draw() {
background(240);
for (let c of circles) {
c.x += c.xSpeed;
c.y += c.ySpeed;
// Bounce off the walls
if (c.x + c.size / 2 > width || c.x - c.size / 2 < 0) {
c.xSpeed *= -1;
}
if (c.y + c.size / 2 > height || c.y - c.size / 2 < 0) {
c.ySpeed *= -1;
}
fill(c.color);
circle(c.x, c.y, c.size);
}
}
function mousePressed() {
circles.push(randomCircleAtPos(mouseX, mouseY));
}
function randomCircleAtPos(x, y) {
return {
x: x,
y: y,
size: random(20, 80),
color: color(random(255), random(255), random(255)),
xSpeed: random(-2, 2),
ySpeed: random(-2, 2)
};
}
There are many niceties provided by p5.js here:
- No need to write any HTML! The createCanvas call will create a canvas element and all subsequent drawing and interaction happens on it [2].
- setup is a "magic" function that gets invoked once at the beginning of the program.
- draw is another magic function that gets automatically called for every animation frame (p5.js arranges the correct requestAnimationFrame calls behind the scenes).
- There are many useful helper functions for drawing, without having to deal with the HTML canvas API, e.g. background, fill, circle. In particular, there's no need to deal with canvas contexts or paths.
- mousePressed is yet another magic function that is called on mouse clicks within the canvas; mouseX and mouseY are magic globals that specify the mouse location within the canvas (without having to worry about client position offsets, etc.)
- Utility functions like color and random have convenient, simple APIs. p5.js has many others, specifically tuned for developing simulations and games. There are vectors, utilities for smooth random noise, functions for mapping between linear ranges, etc.
I also wrote a version of the same animation without p5.js, using plain JS and canvas APIs instead. It's available on GitHub - feel free to compare!
Some history
It all started with Processing, a 25-year-old Java library designed to teach people how to code by creating animations and games (and conversely, giving artists and animators simple tools to enhance their work with code). In 2007, John Resig (of jQuery fame) developed Processing.js - a JS clone of Processing; Khan Academy started using Processing.js for a programming unit on their website.
p5.js was created in 2013; here's a brief history from their GitHub repository:
p5.js was created by Lauren Lee McCarthy in 2013 as a new interpretation of Processing for the context of the web. Since then we have allowed ourselves space to deviate and grow, while drawing inspiration from Processing and our shared community. p5.js is sustained by a community of contributors, with support from the Processing Foundation.
As a result of p5's growth in popularity, Processing.js has been archived a few years ago and new users are directed to p5.js.
While p5.js has a very similar feel to the original Java Processing library, I strongly recommend the former. With the ubiquity of the web these days, there's really no reason to use the Java variant with all the complexity of installation and running separate tools it requires. The browser is all you need!
Educational resources
At the time of writing, Khan Academy's Computer Programming course (starting at unit 4) still uses Processing.js, but it's similar enough to p5.js that I recommend ignoring the difference and just doing it - it's a great resource.
The Coding Train is another fantastic resource that uses p5.js directly to teach programming for beginners in a friendly and engaging style. If you prefer a book format with more advanced material, check out Nature of Code from the same author.
Finally, p5.js comes with its own online editor, where you can create an arbitrary number of projects (each with multiple files, if you want), and have a live preview of everything in the browser.
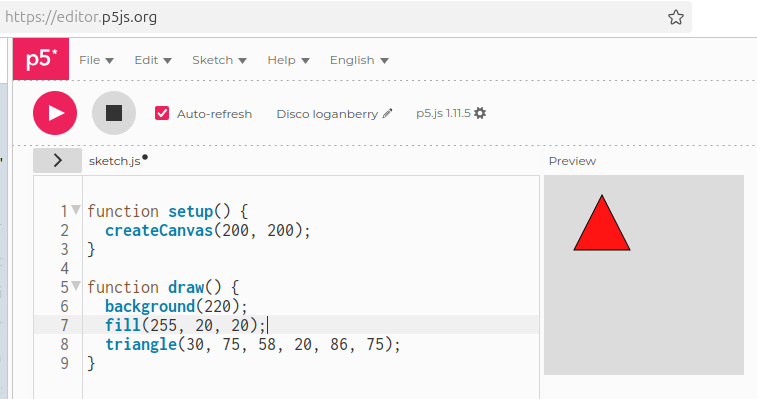
Using p5.js for professional programming?
OK, so p5.js is a great resource for beginners learning to write code. Is it recommended for professional programming, though? Should you incorporate p5.js in your frontend work?
This depends, but in general I'd recommend against it. At the end of the day, it all comes down to the benefits of dependencies as a function of effort. p5.js has a very wide and shallow API - if you're already a seasoned programmer familiar with JS, the additional functionality p5.js provides is fairly trivial [3]. Sooner or later you'll find yourself at odds with p5.js's abstraction or implementation of some concept and will start looking for a way out. For experienced programmers, the raw canvas API isn't that bad to deal with, so the biggest benefits of p5.js dissipate rather quickly.
That said, if you want to hack together a quick game or simulation and p5.js makes your life easier - why not! Just remember the benefit vs. effort curve.
[1] | This sometimes raises eyebrows for experienced programmers, because JavaScript has a certain reputation. My view is that none of this matters for beginners - they typically don't care about our pedantic nuances, and for them JS is as good as any other language. But the environment in which JS executes is the real boon. Imagine you're a kid with a Chromebook; to create a simple game with JS, you don't have to install anything. Just open any web-based JS IDE (e.g. CodePen, JSFiddle, or - better yet - p5.js's own online editor) and start coding. With some honest copy pasting, you can go from blank screen to colorful objects moving around and interacting with your mouse in less than a minute. |
[2] | If you follow the source of my animation on this page, you'll notice I'm cheating a bit - I do need to create an explicit canvas element in HTML because I have to properly embed it within this blog post. But for demos where all you have on the screen is that canvas - this isn't needed. Beginning programmers can completely ignore the existence of HTML and CSS when starting with p5.js! |
[3] | As an example of what I mean, here's p5min - a minimal clone of p5.js sufficient to run the circles demo shown earlier in this post. It's not hard to keep extending it gradually to implement additional functionality, as needed. |