A function that satisfies for its entire domain is called an even function (also sometimes referred to as symmetric). Its graph is symmetric w.r.t. the y axis. Some examples of even functions are and :
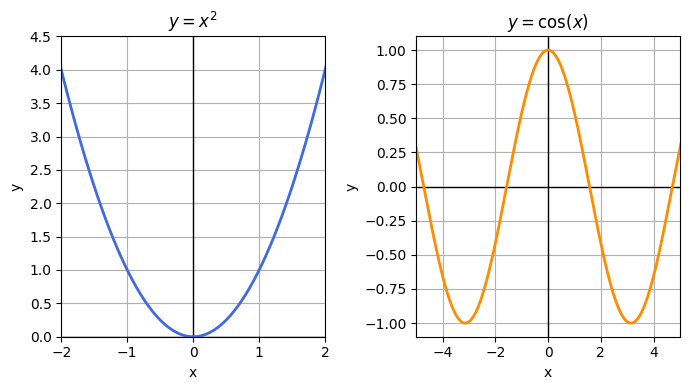
A function that satisfies for its entire domain is called an odd function (also sometimes referred to as anti-symmetric). Its graph is flipped to negative across the y axis. Note that all odd functions must have . Some examples of odd functions are and :
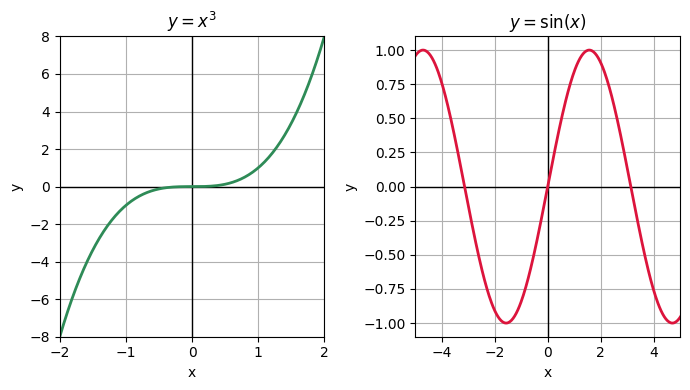
Sums of odd and even functions
The sum of two even functions is even. This is trivial to show: say and both and are even. Then:
So is also even.
Similarly, the sum of two odd functions is odd. If and are odd, then for :
Note, however, that we can’t say much about the sum of and odd and an even function. It can be neither even or odd. Consider , for example. Even though it’s a sum of an even function and an odd function, when we check its own property we get:
This certainly isn’t equal to either or . Such
sums do play a fascinating role later in the post, however.
Products of odd and even functions
It’s similarly easy to show that:
- The product of two even functions is even
- The product of two odd functions is even
- The product of an odd function and an even function is odd
Let’s prove the last statement as an example. We have where is even and is odd.
Integrating even and odd functions over symmetric intervals
Suppose we’re integrating an even function over a
symmetric interval :
We can split the integral into two parts:
Let’s focus on the first part, and rewrite it using the fact that
is even, meaning that :
Now let’s do a variable substitution, . We then have to change the integration bounds and also remember to change to :
We can now reverse the bounds of the last integral, going from 0 to instead of from to 0. Reversing the bounds of a definite integral flips its result, so we get:
But is just a dummy integration variable [1], so we can rename it to
:
In conclusion:
Similarly, we can show that for an odd function :
The process is analogous, except that the first half of the integral ends up being the negative of the second half (because is odd).
These make total sense if we think of definite integrals as the area under the curve of the functions being integrated (and allow for negative areas!)
Every function as a sum of odd and even functions
It turns out that any arbitrary function can be expressed as a sum of an odd function and an even function! While this may initially be surprising, it’s easy to to prove.
Let’s assume that the statement is true, i.e.:
Where is an arbitrary function, is an even
function and is an odd function. We can now manipulate this
expression to find out what and should be to
make it true.
We’ll start by considering and using the even-ness and odd-ness of the addends:
So:
And therefore:
Note that is indeed even for any arbitrary .
Similarly:
And therefore:
This concludes the proof - we’ve found and
that add up to .
[1] | The notion of a dummy or bound variable in Math is very similar to the one in programming languages. See also alpha-conversion in the context of Lambda Calculus. |